Using Python Scripts efficiently on Windows
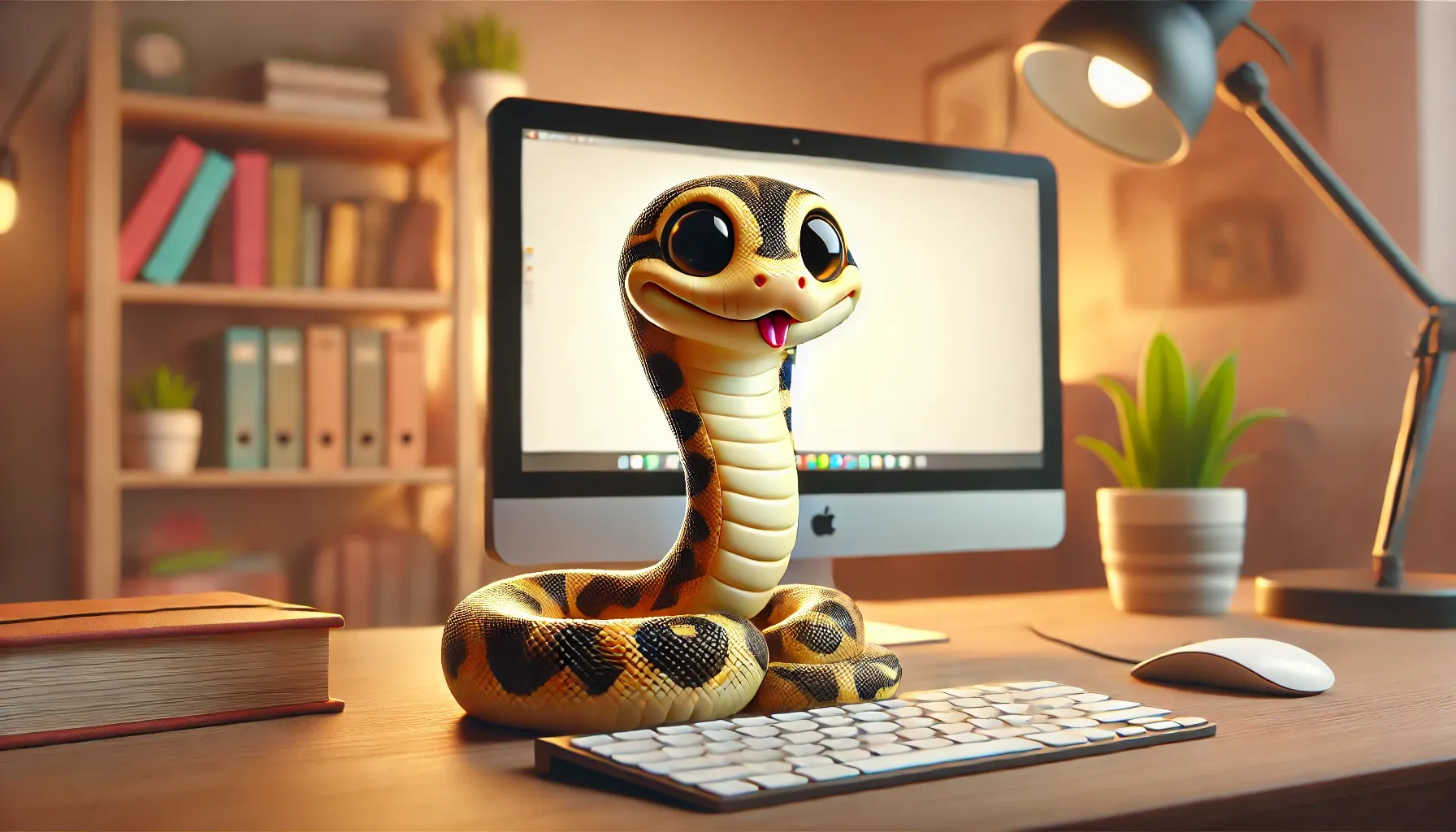
I love writing Python code and recently looked into how I could run my Python scripts in the most efficient way on a Windows machine, optimally indistinguishable from native executables or .cmd / .bat files.
I came up with the following solution, that allows not just that, but if you have multiple versions of Python installed on Windows, set the version to be used in the script itself.
Step 1: Making sure all Python versions are in the Path
Every installed version of Python should be accessible from everywhere via the system path setting. I use Chocolatey to manage Python installations:
choco install python3.9 python3.10 ptyhon3.11 python3.12 -y
After that, make sure, that all of the Python version root and ./Scripts folders are in the path.
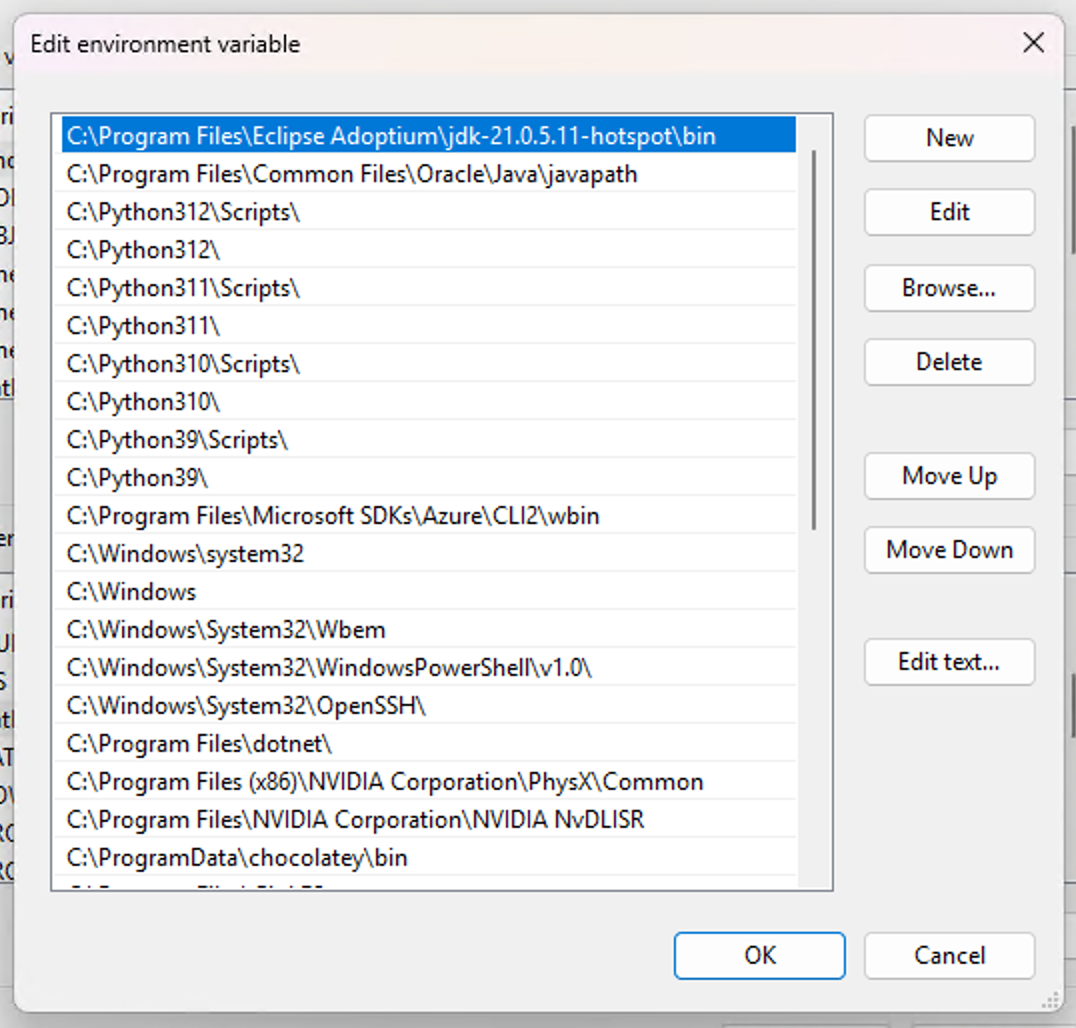
Step 2: Making Windows execute Python scripts without having to type the .py extension
For Windows to properly treat Python files like scripts, you need to add the .py
extension to the PATHEXT system environment variables.
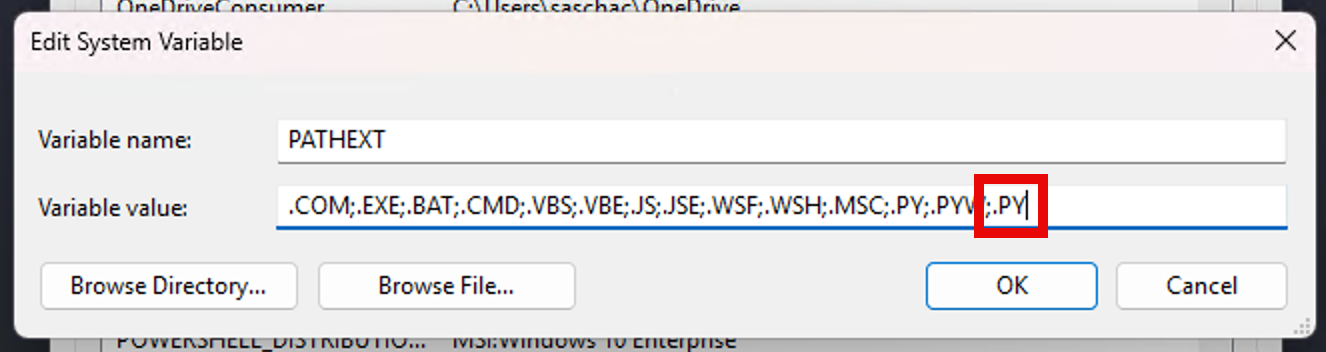
Step 3: Making sure the Python Version Selector is associated with .py files
Python on Windows has an extremely handy tool installed by default, the Python Version Selector py.exe
.
It allows you to selectively run any of the Python interpreters installed on your system, list them, manage them etc.
$ py -3.9 --version
Python 3.9.13
$ py -3.11 --version
Python 3.11.9
$ py -3.12 -m pip install --upgrade pip
(updates PIP in Python 3.12 libraries)
$ py -3.10 -m venv .venv
(creates a new virtual environment based on Python 3.10 in ./.venv)
Just tell Windows to always run .py
files with the Python Version Selector.
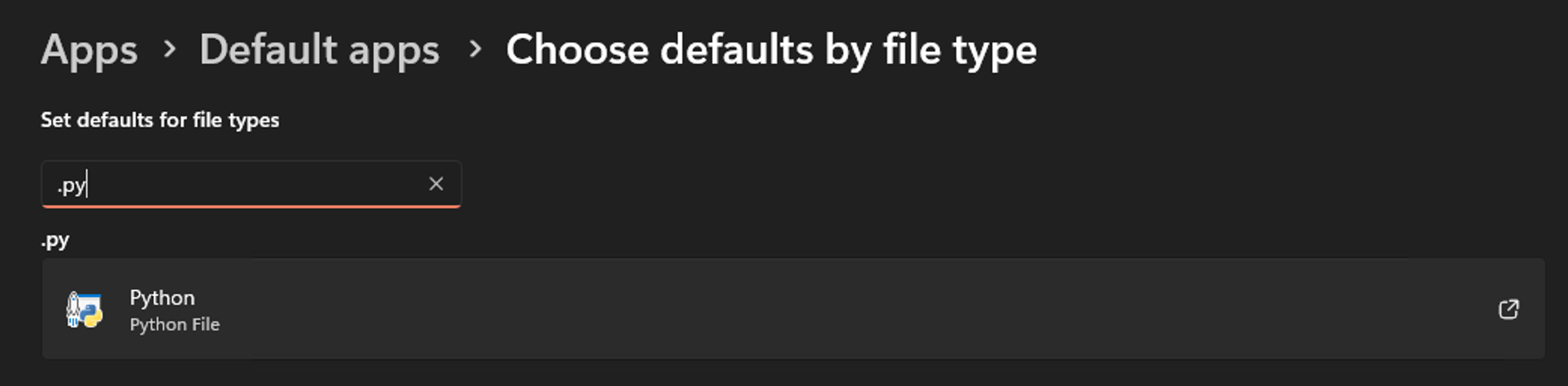
py.exe
is conveniently located in the `C:\Windows
folder.
Step 4: Putting it to the test
Now, let's create a Python script and see if we can run it like any other script. Bonus: We will be selecting the Python version to execute the script right inside the script itself using a shebang.
Create a file python_version.py
and add the following content (select a Python interpreter version that you have installed):
#!/usr/bin/env python3.10
import sys
print(sys.version)
Now, from PowerShell or the command line, just type python_version
and it should run, showing you that it's running Python 3.10:
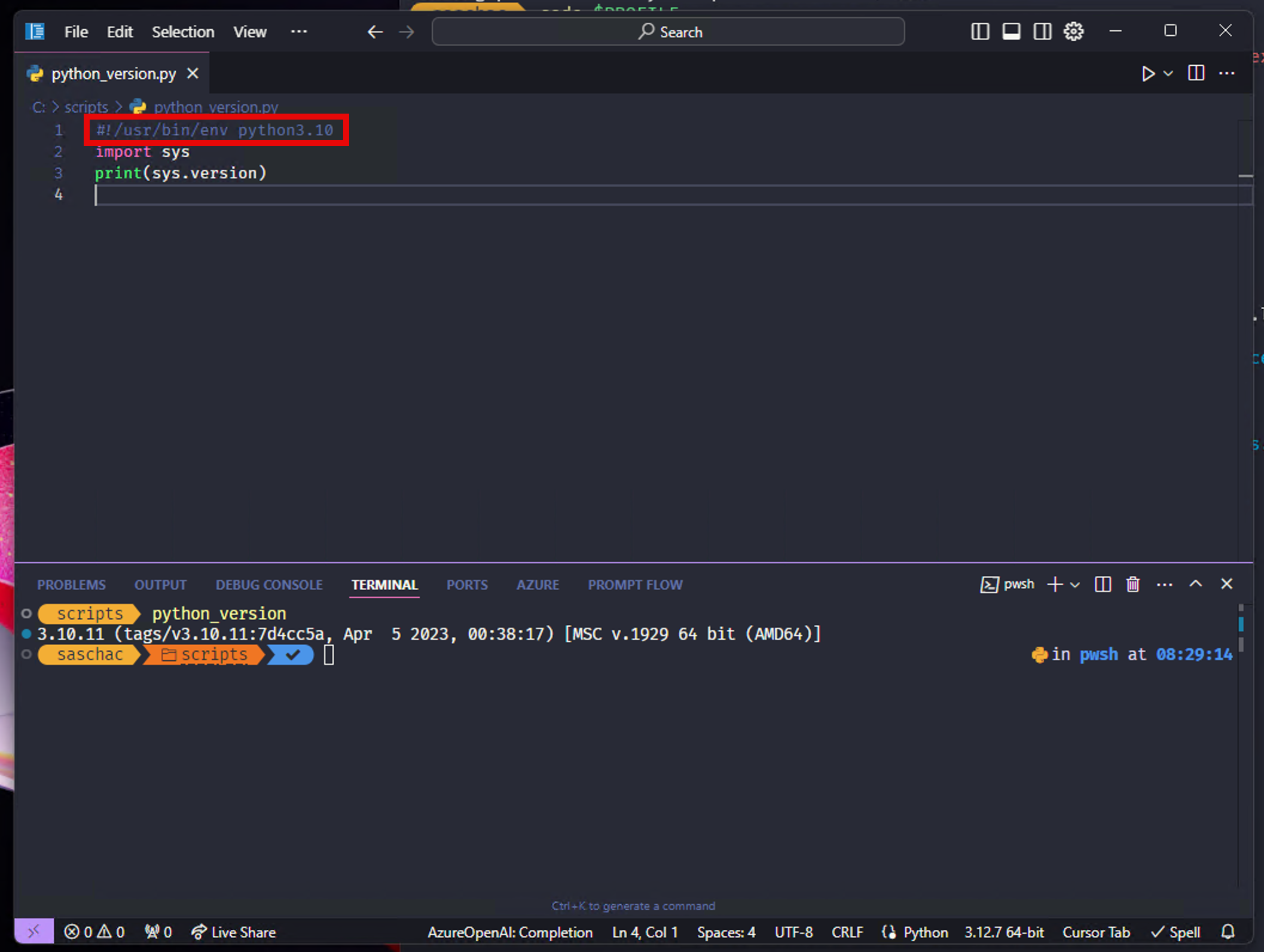
You can now write any* (see caveat below) Python script on your machine like any other script or executable.
The Caveat
If your Python scripts require special PiPY libraries, they will need to be installed system-wide in order for the scripts to work from everywhere on your machine, without having to first activate a virtual environment. This is not necessarily a bad thing, but needs to be "prepared" for the script to work.
To install a Python library globally, you need to have adminitstrative rights, if the Python interpreter you want to target is installed system-wide (i.e. in C:\Python310 instead of C:\Users\YourName\...)
So, to globally install a library in Python 3.10 for example, open PowerShell as Administrator and execute
py -3.10 -m pip install required_library
What about WSL, Linux or Mac?
To do the same in a Unix shell, all you need to do is create the script, give it a filename without an extension (˜/bin/python_version
) and make it executable (chmod +x ˜/bin/python_version
). Voila.